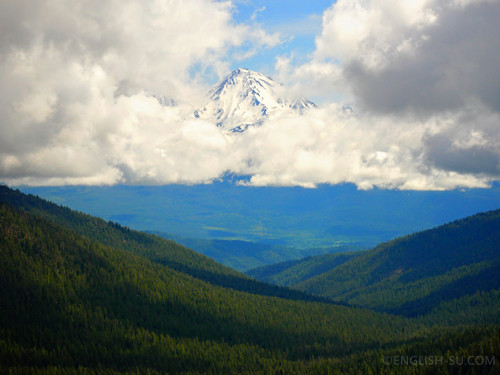
all?
The all?
method in Ruby returns a boolean value (true/false) if all the objects in the list either return true or satisfies the block condition.
Ruby
Enumerable#all?
No block:
>> [ true, "dog", "bear", 19 ].all?
=> true
>> [ nil, true, "hello" ].all?
=> false
With a block:
>> %w(cat dog squirrel bear).all? { |word| word.length >= 3 }
=> true
>> %w(cat dog squirrel bear).all? { |word| word.length <= 3 }
=> false
Elixir
Enum.all?
No block:
>> Enum.all?([ true, "dog", "bear", 19 ])
true
>> Enum.all?([ nil, true, "hello" ])
false
With a block/fn:
>> Enum.all?(["cat", "dog", "squirrel", "bear"], fn word -> String.length(word) >= 3 end)
=> true
>> Enum.all?(["cat", "dog", "squirrel", "bear"], fn word -> String.length(word) <= 3 end)
=> false
Javascript
Array.prototype.every()
Only with callback support:
> [ true, "dog", "bear", 19 ].every(x => x)
true
> [null, true, "hello" ].every(x => x)
false
> ["cat", "dog", "squirrel", "bear"].every(word => word.length >= 3)
true
> ["cat", "dog", "squirrel", "bear"].every(word => word.length <= 3)
false
Read More